Inspector Component
This page is the Part.1 of configuration, we will add the Inspector UI component in your project that provider interaction on browser, like this:
Triggered by hotkeys, also able to be controlled by component props. See the default settings or change them below.
Also provides a panel to show hierarchy from the inspected element to root.
It’s triggered by a right-click when inspecting (version >=v2.1.0
).
Called InspectContextPanel
due to it's like the mouse context-menu
Try it on the Showcase page to see the effect.
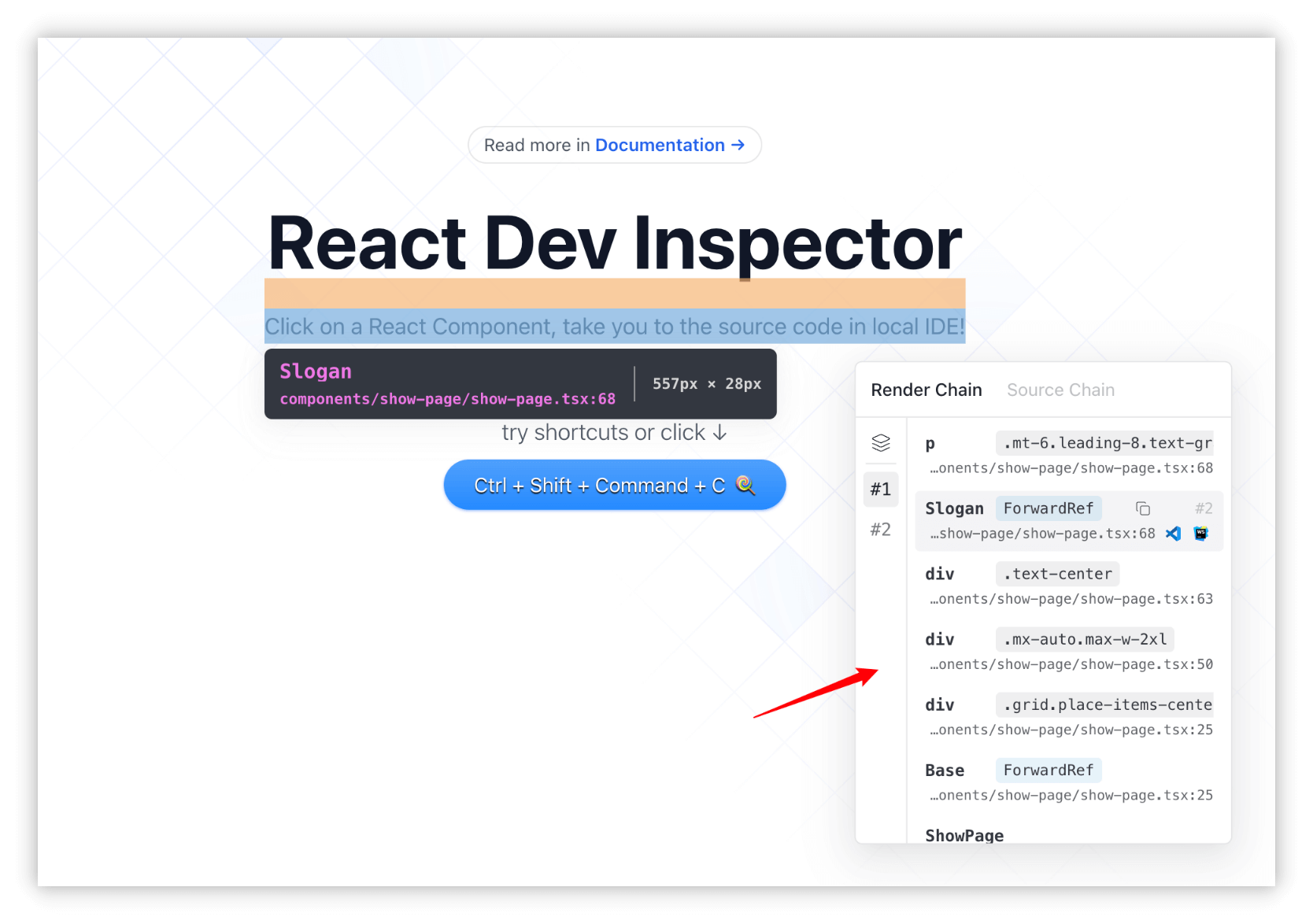
Setup
At first install the package:
npm i -D react-dev-inspector
Then import the react-dev-inspector
in your project entry file, like one of main.tsx
, App.tsx
or index.tsx
:
import { createRoot } from 'react-dom/client'
import { Inspector } from 'react-dev-inspector'
import { App } from './App'
import './index.css'
createRoot(document.getElementById('root') as HTMLDivElement)
.render(
<React.StrictMode>
<Inspector />
<App />
</React.StrictMode>,
)
Now, we've completed the simplest setup way in this Part.1 🎉.
To begin developing your project, you'll gain access to the Inspector UI in the browser via default keyboard shortcuts:
Ctrl + Shift + Command + C
on macOSCtrl + Shift + Alt + C
on Windows / Linux
By default, clicking inspected element in the browser will trigger the <Inspector>
to send an API network request to dev-server,
that will call your local IDE/editor to open the source file.
For the next Part.2, you'll need to do set up a Middleware on Server-Side to handle these API requests.
Certainly, you also have the option to override these shortcuts by configuring the props of the <Inspector>
component.
For more advanced controls, refer to the Props section below.
Props
This section refers to the interface of InspectorProps
(opens in a new tab)
for <Inspector>
component.
<Inspector
keys={...}
onInspectElement={...}
onHoverElement={...}
onClickElement={...}
active={...}
onActiveChange={...}
disable={...}
/>
keys
- Type:
keys?: string[] | null
- Default:
['Ctrl', 'Shift', 'Command', 'C']
on macOS
['Ctrl', 'Shift', 'Alt', 'C']
on other platforms
Activity toggle hotkeys for <Inspector>
component, supported keys see: https://github.com/jaywcjlove/hotkeys#supported-keys (opens in a new tab)
Setting keys={null}
explicitly means that disable use hotkeys to trigger it.
active
Added in: v2.0.0
Type: active?: boolean
If setting active
prop, the Inspector will be a Controlled React Component,
you need to control the true
/ false
state to active the Inspector.
If not setting active
prop, this only a Uncontrolled component that
will activate/deactivate by hotkeys.
onActiveChange
Added in: v2.0.0
Type: onActiveChange?: (active: boolean) => void
Callback trigger by active
state change, includes:
- hotkeys toggle, before activate/deactivate Inspector
- Escape / Click, before deactivate Inspector
Note:
onActiveChange
will NOT trigger by change ofactive
prop key.
disable
Added in: v2.0.0
Type: disable?: boolean
Whether to disable all behavior include hotkeys listening or trigger.
Note: react-dev-inspector
will automatically disable in production environment by default.
inspectAgents
Added in: v2.1.0
- Type:
inspectAgents?: InspectAgent<Element>[]
interface
InspectAgent
(opens in a new tab) - Default: built-in
[new DomInspectAgent()]
Agent for get inspection info in different React renderer with user interaction.
onInspectElement
Added in: v2.0.0
- Type:
onInspectElement?: (params: OnInspectElementParams<Element>) => void
interface
InspectParams
(opens in a new tab)) - Default: built-in
gotoServerEditor
(opens in a new tab) util function
Callback when left-clicking on an element, with ensuring the source code info is found,
the default gotoServerEditor
callback will request the dev-server to open local IDE/editor from server-side.
Note: By override the onInspectElement
prop, the default gotoServerEditor
will be removed,
that means you want to manually handle the source info, or handle how to goto editor by yourself.
You can also use the built-in gotoServerEditor
util funtion in onInspectElement
to get origin behavior (open local IDE on server-side),
it looks like:
import { Inspector, gotoServerEditor } from 'react-dev-inspector'
<Inspector
onInspectElement={({ codeInfo }) => {
... // your processing
gotoServerEditor(codeInfo)
}}
/>
Or simply use other built-in utils likes gotoVSCode
, gotoVSCodeInsiders
, gotoWebStorm
to open IDE/editor by URL-scheme,
which not need any server side configuration:
import { gotoVSCode, gotoVSCodeInsiders, gotoWebStorm } from 'react-dev-inspector'
<Inspector
onInspectElement={gotoVSCode}
// onInspectElement={gotoVSCodeInsiders}
// onInspectElement={gotoWebStorm}
/>
onHoverElement
Type: onHoverElement?: (params: InspectParams) => void
Callback when hovering on an element
onClickElement
Type: onClickElement?: (params: InspectParams) => void
Callback when left-clicking on an element.
children
Type: children?: ReactNode
Any children of react nodes, to support usages like both of:
<Layout>
<Inspector />
<App />
</Layout>
and
<Layout>
<Inspector>
<App />
</Inspector>
</Layout>
disableLaunchEditor
Deprecated after: v2.0.0
- Type:
disableLaunchEditor?: boolean
- Default:
true
if settingonInspectElement
callback, otherwise isfalse
.
Whether to disable default behavior: "open local IDE when click on component".
Deprecated
disableLaunchEditor
is deprecated after v2, please use onInspectElement
callback instead for fully custom controlling.